Like most UX-driven developers, I’m curious to see which front-end JavaScript frameworks live up to the hype. As a plain JavaScript SDK, KeyLines plays nicely with whichever front-end frameworks and components you prefer. That includes emerging technologies, like the breakout star of the State of JavaScript 2022 survey: Svelte. I tried it for myself and wanted to share what I’d learned, so here’s a Svelte tutorial on how to integrate with KeyLines to build a simple graph visualization app.
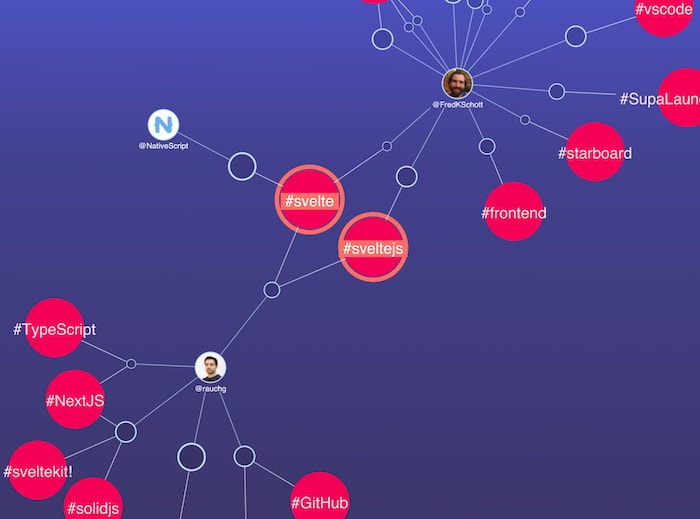
Svelte: cybernetically enhanced web apps
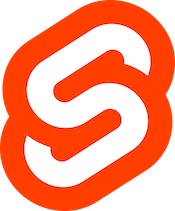
The Svelte JavaScript framework’s goal is to help developers create super-fast web apps through a radical new approach to building user interfaces. Traditional frameworks like React and Vue use high-overhead tasks in the browser to manage things like virtual DOMs, but Svelte uses a more detailed method to identify updates. As a result, it avoids having to do page-wide diffing, reducing the overall workload.
What sets Svelte apart from most other frameworks is its approach to a user’s experience. It aims for the smallest runtime possible, pushing as much work as possible into builds. It doesn’t send as much stuff to the browser, so the browser doesn’t have as much to do. And doing less means faster web apps.
Svelte’s popularity has grown steadily since its release in 2016. It was voted ‘most loved framework’ in Stack Overflow’s 2021 survey, and it just received further accolades with the release of the State of JS 2022.
What the State of JS 2022 said about Svelte
The latest State of JavaScript 2022 survey was their biggest yet, with almost 40,000 responses from developers across the JavaScript community. Of those, almost 30,000 respondents said they used JavaScript for front-end development, which gives us comprehensive feedback on every framework from stalwarts like React to newbies like Solid.
If you’ve read the survey results already, you’ll know Svelte was ranked as the top front-end framework that most survey respondents want to learn. It also ranked high on retention: 90% of respondents who’ve used it before are keen to use it again.
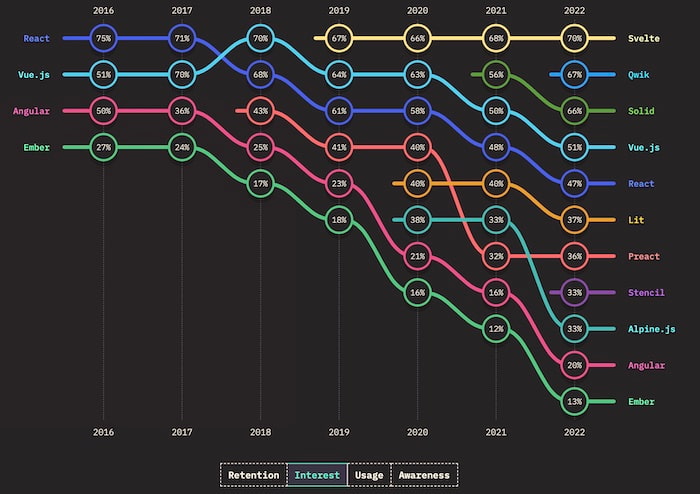
Svelte tutorial: how to build a KeyLines app
To get to grips with Svelte, let’s create a basic KeyLines graph visualization app that:
- has data that we can modify at runtime (because nobody uses hardcoded data)
- includes event handlers – we’ll just add a context menu
- can compute new downstream values using bits of state
Use the Svelte wrapper
First, I wrote a thin Svelte wrapper for KeyLines:
<script context="module"> import KeyLines from '../node_modules/keylines/esm/index.js'; let componentIndex = 1; </script> <script> // essential component functionality import { onDestroy, onMount, createEventDispatcher } from 'svelte'; const dispatch = createEventDispatcher(); export let containerId; let componentId; let chart; // pass events up to the parent function onEvent(props) { dispatch(props.name, props.event); } // form the DOM id to use if one is to be created const actualContainerId = containerId || `KeyLines-chart-wrapper-${componentIndex || 1}`; onMount(async () => { componentId = componentIndex; componentIndex++; KeyLines.create({container: actualContainerId, options}).then((loadedChart) => { passComponentReference(actualContainerId, loadedChart); chart = loadedChart; chart.options(options); chart.on('all', onEvent); if (items !== undefined) { chart.load({ type: 'LinkChart', items }); chart.layout(undefined, { consistent: true, fit: view === undefined }); } if (view !== undefined) { chart.viewOptions(view); } }); }); onDestroy(() => { if (chart) chart.destroy(); }) // return a reference to the chart object so the parent can access all API methods if they want function passComponentReference(id, componentRef) { dispatch('componentCreated', { id, component: componentRef}); } </script> <div id={actualContainerId} style='width: 100%; height:100%;'/>
The first thing I notice about Svelte is that it’s essentially HTML. This is surprising at first because other frameworks tend to migrate away from HTML and represent components in terms of JavaScript objects (think React classes and functional components). But for practical reasons, this decision makes sense. We’re creating DOM elements and we’re varying them using JavaScript code that we put into script tags. So Svelte just embraces this pattern for .svelte files.
Svelte defines both internal values and externally supplied props in a minimal way:
export let containerId; let componentId; let chart;
Variables are defined as if you were writing in standard JavaScript, and any variable is persisted. To update the value, you just set it to something else and Svelte reactively updates the parts of the component that need changing: no special management needed. Props are just as intuitive, with Svelte leveraging the export keyword to define them.
Add event handlers
Svelte doesn’t need you to define events in your component. You just have to use the dispatch returned from the built in createEventDispatcher to fire them.
// pass events up to the parent function onEvent(props) { dispatch(props.name, props.event); } ... chart.on('all', onEvent);
Here we use KeyLines’ ‘all’ event to pass any event KeyLines charts fire straight out of the component, so that any attached handlers can run. In our app component, we’ll store the information about the item the context menu event was fired on:
on:click={(event) => { showContextMenu = event.id !== null; currentContextMenuItem = event.item; }}
Set up computed values
In the final step of this Svelte tutorial, we’ll set up values to display the number of items in our data in a counter.
In a render-based setup, we’d have to throw these values into the root of our component or render function, but with Svelte, we add the $ label using the JS Label Syntax.
Svelte interprets these labels as the definition of a variable that is reactive, which means it depends on the values used to calculate it. Svelte then optimizes the runtime by automatically detecting which variables are required to create it. It watches for changes in the values and updates only when needed, which is really handy.
$: numberOfItems = items.length ... <div style='position: absolute; right: 10px;'>There are {numberOfItems} items in the chart<div>
A nice little extra (which KeyLines already handles itself) is module-level code. If you use more than one KeyLines chart, it’s a good idea to keep tabs on each of the contexts, and Svelte’s module script tag does this really neatly.
<script context="module"> import KeyLines from '../node_modules/keylines/esm/index.js'; let componentIndex = 1; </script>
Svelte tutorial complete – what’s your verdict?
I can see why Svelte has a high level of satisfaction and gets so much developer interest. Its creators really thought about the issues with previous frameworks and how to best address them. The code is left much cleaner than the likes of React, Angular or Vue, with little to no boilerplate. The syntax is more intuitive too. It also features comparatively smaller builds, which creates code that’s faster to get to users and quicker to run.
Svelte is a nice step in the right direction, although you may prefer to wait until it breaks into the production toolchains in a big way before you throw away your React app. Svelte’s current range of supporting tools is limited compared with other frameworks, and their community is much smaller. I think it suffers a little from locking you into certain infrastructure choices, but I’m sure the future will bring packages to solve these issues.
Have you used Svelte yet? Why not run through this tutorial and let us know what you think. If you’re not using KeyLines already, simply sign up for a free trial.