Our article on how to build a React graph visualization app using Styled Components is one of our most popular developer guides. In this Tailwind CSS tutorial, we’ll show you another way to customize your ReGraph app.
What is Tailwind CSS?
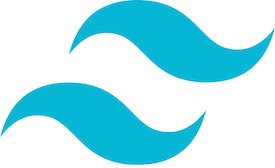
Tailwind CSS is a utility-first CSS framework for creating highly-customized user interfaces fast.
Relying on utility classes makes it easier to apply design elements consistently and build responsive designs – there’s no need for masses of CSS. It also comes with sensible off-the-shelf style defaults to get started quickly, but you’ll want to take advantage of the many customization options.
Why use Tailwind CSS with ReGraph?
Developers choose ReGraph, our graph visualization toolkit, because it’s the best toolkit for building high-performance graph visualizations to add to their React applications.
The ability to customize every element, interface, interaction and workflow means they can create the exact design their users want. There’s also a live-coding playground to try out design ideas and share them with teammates.
This approach sits well with Tailwind CSS users. They too benefit from fast prototyping, virtually limitless customization options, and attractive color schemes for beautiful, eye-catching interfaces.
I’ll walk you through the best way to use Tailwind CSS and ReGraph to create something special.
About the insurance fraud dataset
For our Tailwind CSS tutorial we’ll use an extract from a fictitious vehicle insurance claim dataset. To see the full insurance fraud investigation in action, read Designing simple visual models that empower & delight users
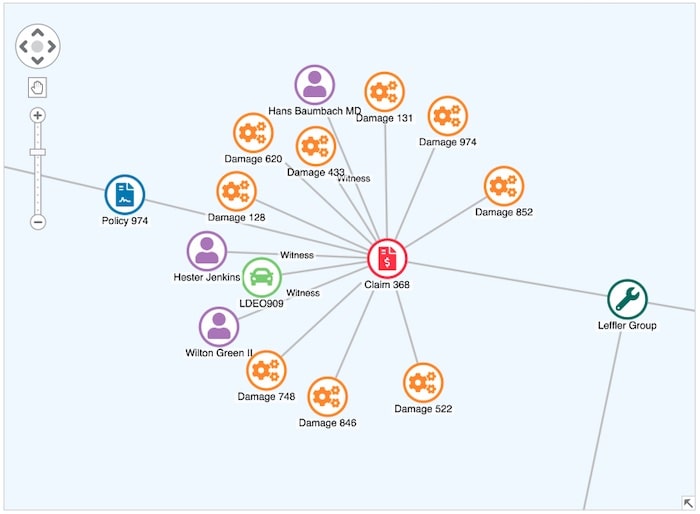
Our data connects nodes representing mechanics with the repairs they carried out on damaged vehicles. Some damage – like that caused by rear-end collisions – is far more common, but you’d also expect a range of repairs across different vehicles.
If a mechanic claims they fixed an unusually high number of issues affecting one specific vehicle part, it could be a sign of claim inflation. This is a big problem in the automotive insurance industry: policyholders conspire with fraudulent mechanics to claim for more damage than actually occurred. We’ll visualize our dataset and see what insights we can uncover.
Our data model lists how many times each mechanic fixed a specific vehicle part:
[ { "mechanic": "Aufderhar LLC", "type": "Driver's Front Door", "count": 6 }, ... { "mechanic": "Fraser's", "type": "Driver's Rear Door", "count": 14 }, ... { "mechanic": "Thiel's Auto", "type": "Wing Mirror", "count": 4 } ]
We’ll save the data as src/data.json ready to import later.
Get started with ReGraph
The begin our Tailwind CSS tutorial, we create an empty React application using the create-react-app command:
yarn create react-app tailwind-css-demo cd tailwind-css-demo
We include the latest version of ReGraph, downloaded from the ReGraph SDK site:
cp ~/Downloads/regraph-[version].tgz . yarn add ./regraph-[version].tgz
With ReGraph installed, we can create our first simple visualization of the data. We define a function to convert the data from the data.json file into ReGraph links and nodes. We also define two functions – styleNode and styleLink – to create ReGraph node and link objects respectively.
The link widths will scale according to the number of times a mechanic billed for repairs to a particular vehicle part.
Replace the contents of file src/App.jsx with:
import { Chart } from "regraph"; import data from "./data.json"; function styleNode(text) { const label = { text, center: false, }; return { label }; } function styleLink(id1, id2, count) { const width = count * 0.4; return { id1, id2, width }; } function convertData() { const items = {}; data.forEach((entry) => { const { mechanic, type, count } = entry; if (!(mechanic in items)) { items[mechanic] = styleNode(mechanic); } if (!(type in items)) { items[type] = styleNode(type); } items[`${mechanic}-${type}`] = styleLink(mechanic, type, count); }); return items; } export default function App() { return <Chart items={convertData(data)} />; }
We’ve done enough already to create our first basic graph visualization:
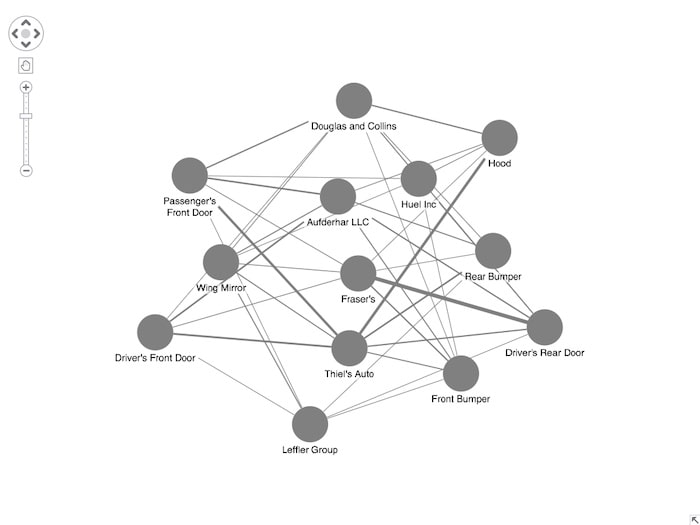
Even from this basic view, we can see from the wider links how some mechanics carried out more repairs to certain vehicle parts compared to others. That’s a useful start.
Adding Tailwind CSS to the app
Next we install Tailwind CSS to the app by following the steps in the excellent Tailwind CSS documentation.
First we add Tailwind CSS, PostCSS (a tool for transforming CSS with JavaScript) and CRACO (to override create-react-app configuration easily) to the app by running:
yarn add -D tailwindcss@npm:@tailwindcss/postcss7-compat postcss@^7 autoprefixer@^9 @craco/craco
Then we update the package.json file to start the application through CRACO instead of create-react-app.
"scripts": { - "start": "react-scripts start", - "build": "react-scripts build", - "test": "react-scripts test", + "start": "craco start", + "build": "craco build", + "test": "craco test", "eject": "react-scripts eject" }
We also create a craco.config.jsfile to enable the Tailwind CSS and Autoprefixer plugins for PostCSS:
module.exports = { style: { postcss: { plugins: [require("tailwindcss"), require("autoprefixer")], }, }, };
Once this is done, we generate the tailwind.config.js file in the root of our project:
npx tailwindcss-cli@latest init
After the file is created, we create a symbolic link to it from the src/ directory of the project. We’ll use this later to import the configuration file from the code of our application.
cd src/ ln -s ../tailwind.config.js ./
We’ll also update the src/index.css file to:
- load the styles generated by Tailwind into our app
- apply the h-screen rule to the React root element so that the app will always cover the entire height of the visible window
-body { - margin: 0; - font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', 'Roboto', 'Oxygen', - 'Ubuntu', 'Cantarell', 'Fira Sans', 'Droid Sans', 'Helvetica Neue', - sans-serif; - -webkit-font-smoothing: antialiased; - -moz-osx-font-smoothing: grayscale; -} +@tailwind base; +@tailwind components; +@tailwind utilities; -code { - font-family: source-code-pro, Menlo, Monaco, Consolas, 'Courier New', - monospace; +#root { + @apply h-screen; }
Finally, to restart our app to redisplay our basic insurance fraud visualization, run:
yarn start
Accessing Tailwind colors from our application
With Tailwind added to our application, we can now access its configuration from our code.
To do this, let’s import the function resolveConfig and the tailwind.config.js file (we linked this into the <src/ directory earlier) into our app:
import { Chart } from "regraph"; +import resolveConfig from "tailwindcss/resolveConfig"; import data from "./data.json"; +import tailwindConfig from "./tailwind.config.js"; + +const { theme } = resolveConfig(tailwindConfig);
With those imported, we can use Tailwind CSS’s theme configuration in our app, just like in the code above. This gives us access to values such as colors (i.e. theme.colors.green[500]) or sizes (i.e. theme.fontSize.base for the base font size). Defaults are inherited from Tailwind’s standard settings.
Next we update our styleNode function. We add a new parameter type to it to control the node colors:
- green-500 (#10B981) for mechanics
- blue-500 (#3B82F6) for damage types
We update the labels to use gray-700 (#374151) for the text against a transparent background.
-function styleNode(text) { +function styleNode(text, type) { + const color = type === "mechanic" ? theme.colors.green[500] : theme.colors.blue[500]; const label = { text, + color: theme.colors.gray[700], + backgroundColor: theme.colors.transparent, center: false, }; - return { label }; + return { color, label }; } function styleLink(id1, id2) {
For the two call sites of styleNode to pass in the correct node type (mechanic or damage) we use:
data.forEach((entry) => { const { mechanic, type, count } = entry; if (!(mechanic in items)) { - items[mechanic] = styleNode(mechanic); + items[mechanic] = styleNode(mechanic, "mechanic"); } if (!(type in items)) { - items[type] = styleNode(type); + items[type] = styleNode(type, "damage"); } items[`${mechanic}-${type}`] = styleLink(mechanic, type, count); }); return items; }
We also update the chart’s background color to gray-50 (#F9FAFB).
export default function App() { - return <Chart items={convertData(data)} />; + return <Chart items={convertData(data)} options={{ backgroundColor: theme.colors.gray[50] }} />; }
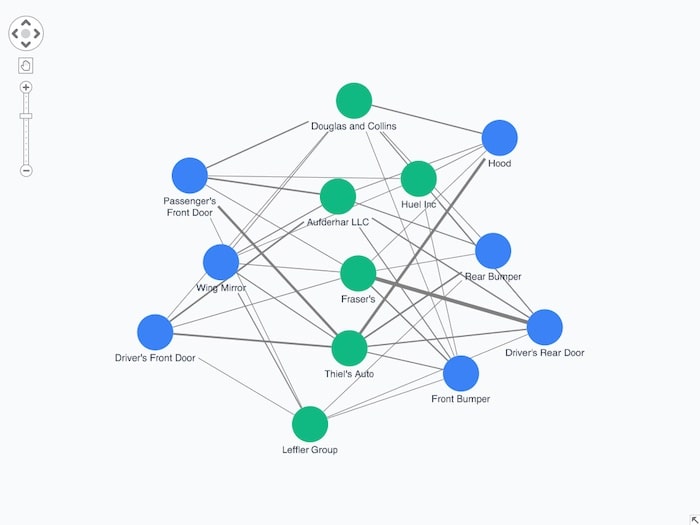
Link styling to reveal hidden patterns
So far we’ve successfully set the node colors in our Tailwind CSS tutorial. Now we’ll turn our attention to styling links. Remember, each link represents how often a mechanic claimed to have fixed damage to a specific vehicle part.
Fraud analysts have to make fast decisions about which areas of their investigation to prioritize. To make the most unusual activities stand out, we’ll color the links differently depending on how many times repairs were carried out to that part of any vehicle:
- one to five times = we’ll make the links grey-500 (#6B7280)
- six to ten times = we’ll make the links yellow-500 (#F59E0B)
- more than ten times = we’ll make the links red-500 (#EF4444)
We’ll also turn the orange and grey links semi-transparent as they’re potentially less important.
Tailwind’s colors are in hexadecimal format. To change the opacity, we’ll use functions from the tinycolor2 package, a handy JavaScript library for manipulating colors and converting them to different formats.
First we install the library:
yarn install tinycolor2
Next we add the package to the list of our imports in App.js.
import { Chart } from "regraph"; import resolveConfig from "tailwindcss/resolveConfig"; +import tinycolor from "tinycolor2"; import data from "./data.json"; import tailwindConfig from "./tailwind.config.js";
Now that the package is imported as tinycolor, we can use it with the styleLink function. The process is:
- call the package with the colors whose alpha values we want to change
- call the setAlpha function to turn the color semi-transparent
- convert it back to a string by calling .toRgbString
function styleLink(id1, id2, count) { + const width = count * 0.4; + let color = tinycolor(theme.colors.gray[500]).setAlpha(0.4).toRgbString(); + if (count > 10) { + color = theme.colors.red[500]; + } else if (count > 5) { + color = tinycolor(theme.colors.yellow[500]).setAlpha(0.8).toRgbString(); + } + return { id1, id2, width, color }; }
Now our graph visualization features styled links as well as colored nodes:
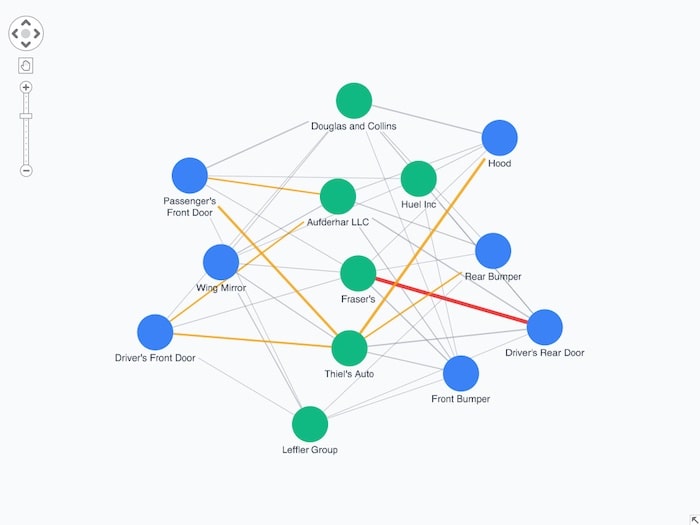
We get a clearer view of which mechanics claimed for work on a specific vehicle part more than five times, and one in particular – Fraser’s – who allegedly repaired more than ten driver’s rear doors. Suspicious?
Adding icons to the mix
Familiar icons make nodes instantly recognizable. We’ll use the excellent Font Awesome icon set to show mechanics as a wrench icon (fa-wrench) and damaged vehicle parts as a set of cogs (fa-cogs).
We install Font Awesome icons with:
yarn add @fortawesome/fontawesome-free
Then we import it into our app. At the same time, we import ReGraph’s FontLoader component.
-import { Chart } from "regraph"; +import { Chart, FontLoader } from "regraph"; import resolveConfig from "tailwindcss/resolveConfig"; import tinycolor from "tinycolor2"; import data from "./data.json"; import tailwindConfig from "./tailwind.config.js"; +import "@fortawesome/fontawesome-free/css/fontawesome.css"; +import "@fortawesome/fontawesome-free/css/solid.css";
Next we update our App function to wrap the Chart component in the FontLoader component. This makes sure that the chart is only rendered once the “Font Awesome 5 Free” font is loaded. We also configure the chart to use this as the default for any font icons we set.
- return <Chart items={convertData(data)} options={{ backgroundColor: theme.colors.gray[50] }} />; + return ( + <FontLoader config={{ custom: { families: ["Font Awesome 5 Free"] } }}> + <Chart + items={convertData(data)} + options={{ + backgroundColor: theme.colors.gray[50], + iconFontFamily: "Font Awesome 5 Free", + imageAlignment: { "fa-cogs": { size: 0.8 }, "fa-wrench": { size: 0.8 } }, + }} + /> + </FontLoader> + );
Lastly, we update the styleNode function. This sets the correct font icon based on the type of node: fa-wrench for mechanics and fa-cogs for damage types.
- return { color, label }; + const fontIcon = { + color: theme.colors.white, + text: type === "mechanic" ? "fa-wrench" : "fa-cogs", + }; + return { color, fontIcon, label };
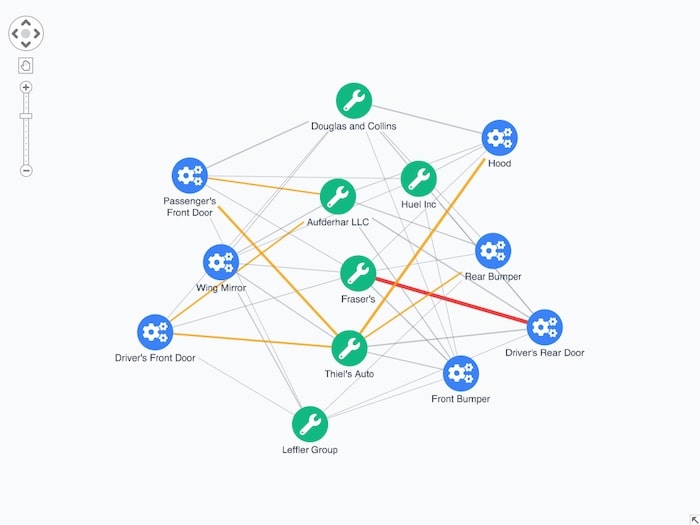
Practical context menus
A powerful way to provide useful details about the chart without overcrowding it is by adding clever interactions. Here, we trigger an onContextMenu event by right-clicking on Fraser’s to reveal exactly how many repairs they’ve claimed for.
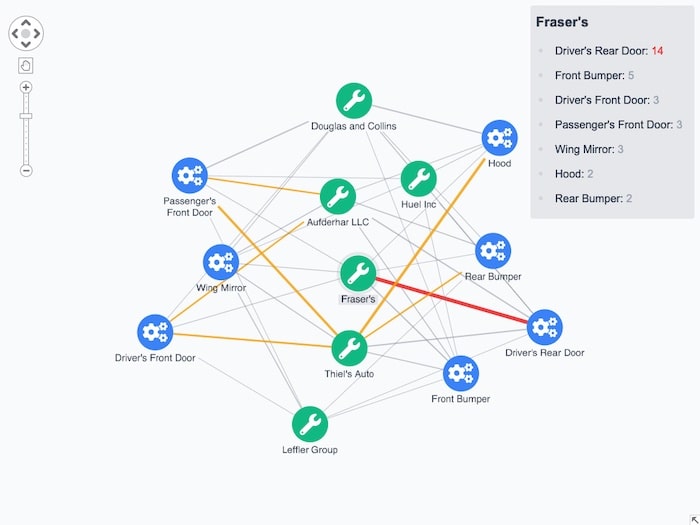
For more on this, check out 4 easy styling options for interactive graph visualization
Easy theming to turn light into dark
If you want graph visualizations styled in dark mode for improved accessibility or better esthetics, it’s easy to achieve with ReGraph and Tailwind CSS.
Using Tailwind’s ‘dark’ variant and ReGraph’s controlTheme option, you can apply a dark theme with matching navigation and controls.
Get started with ReGraph & Tailwind CSS
I hope you found this ReGraph and Tailwind CSS tutorial useful! We’ve kept things simple here, but the scope and variety of styling options available from both libraries are huge.
If you’re ready to start designing beautiful graph visualization apps, request a free trial