Remix and ReGraph, our graph visualization toolkit, are a match made in heaven when it comes to quickly building React apps. In this technical tutorial for developers, we’ll show you how a simple mixture of wraps and hooks in TypeScript gets ReGraph running smoothly in this full-stack web framework.
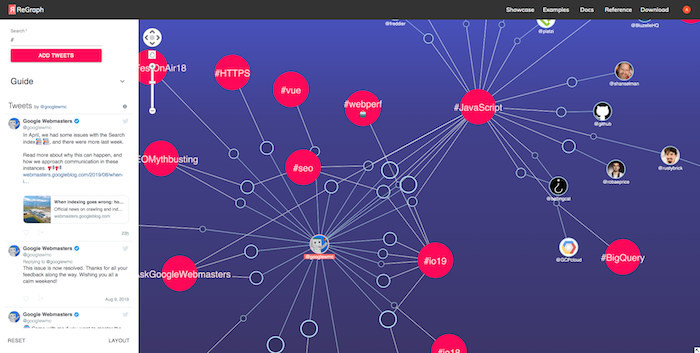
What is Remix?
Remix is a JavaScript framework for modern UX. It takes a new approach to building React web applications, empowering users to switch seamlessly between front end and back end code – and its nested routing gives it an edge over Next.js.
If you need fast results, you’ll enjoy working with Remix because it’s designed to take care of the heavy lifting in website and web app development. All you have to worry about is choosing the best building blocks to meet your needs. And if you need a powerful visualization component – check out ReGraph.
Not using ReGraph already?
You’ll need it to follow this Remix tutorial.
Why ReGraph is a good fit for a Remix project
ReGraph is a natural partner for Remix, which comes from the creators of React Router, UNPKG and Reach UI. It’s designed for building scaleable, accessible, high-performing React apps quickly, and we share Remix’s commitment to offering an intuitive (and enjoyable) UX.
Both ReGraph and Remix are well supported with guides, tutorials and playgrounds. When you combine our fully customizable component with the flexible Remix framework, the possibilities for your React project are endless.
Bringing Remix and ReGraph together
Like Next.js, Remix provides both client and server-side rendering, which can catch ReGraph unawares. Unlike conventional React components, ReGraph needs various DOM APIs to function – which it won’t get on the server side.
But that doesn’t mean Remix and ReGraph can’t work together. We can keep ReGraph in its comfort zone by wrapping its components, to keep them client-side.
We do this using TypeScript code. Make sure you have TypeScript 3.8 or above, because we’ll rely on the “import type” functionality, which isn’t supported by earlier iterations.
Let’s start with the basics:
import { useEffect, useState } from 'react'; import type { Chart as RegraphChart, TimeBar as RegraphTimeBar, FontLoader as RegraphFontLoader, } from 'regraph'; type RegraphModuleType = typeof import('regraph'); let regraphModulePromise: Promise<{ default: RegraphModuleType }> | undefined;
Hooks in your ReGraph code save a lot of time and effort. In this example we’re using a hook to dynamically load the ReGraph module at runtime. We also add code to make sure that:
And when it’s loaded, we store it in state. Another hook triggers a re-render of the wrapper components.
function useLoadRegraph() { const [regraph, setRegraph] = useState(); useEffect(() => { if (regraphModulePromise == null) { regraphModulePromise = import('regraph'); } regraphModulePromise.then((module) => setRegraph(module.default)); }, []); return regraph; }
Now we add a simple wrapper for the chart component. We also want to load the ReGraph library, making sure that it doesn’t render before it’s fully loaded.
export function Chart(props: RegraphChart.Props) { const regraph = useLoadRegraph(); if (regraph == null) { return null; } return <regraph.Chart {...props} />; }
When that’s done, we forward any props, and add wrappers for the TimeBar and FontLoader – as we did for the chart.
export function TimeBar(props: RegraphTimeBar.Props) { const regraph = useLoadRegraph(); if (regraph == null) { return null; } return <regraph.TimeBar {...props} />; } type RegraphFontLoaderProps = React.PropsWithChildren< React.ComponentPropsWithoutRef<typeof RegraphFontLoader> >; export function FontLoader(props: RegraphFontLoaderProps) { const regraph = useLoadRegraph(); if (regraph == null) { return null; } return <regraph.FontLoader {...props} />; }
And that’s a wrap!
This simple bit of code means that Remix’s seamless server and browser runtime environment now fits ReGraph like a glove. The ReGraph chart can load from anywhere in your app, whether the request is rendered client- or server-side. We’ll save the data as app/components/regraph.tsx ready to import. Here’s the code for a sample chart:
import { Chart } from '~/components/regraph'; export default function Index() { return ( <Chart items={{ a: { color: '#75b3ff', glyphs: [{ angle: 180, label: { text: 'Hello from ReGraph' }, radius: 40 }], label: { text: '💿', backgroundColor: 'transparent', fontSize: 40 }, size: 3.5, }, }} options={{ backgroundColor: '#1e1e1e', controlTheme: 'dark', iconFontFamily: 'Font Awesome 5 Free', }} /> ); }
And here’s how that chart will look:
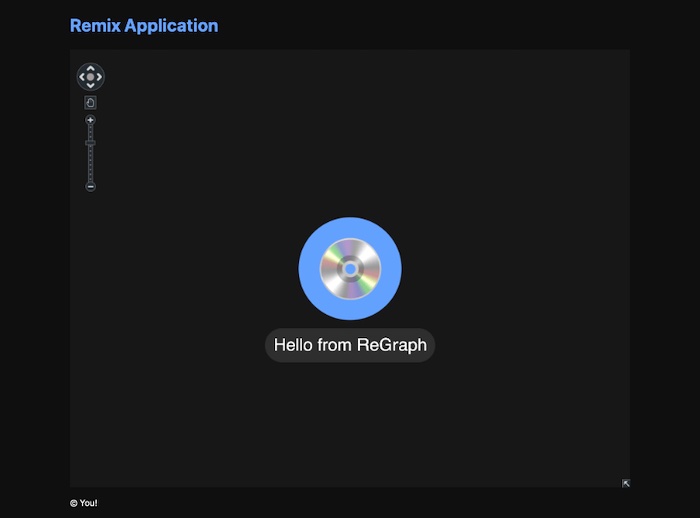
This example is pretty minimalist in style – but when it comes to customizing your apps, there are lots of options out there. Check out how to use ReGraph with Styled Components or Tailwind CSS.
Try out ReGraph & Remix
Remix is open source, so if you grab yourself a free trial of ReGraph, you can try both tools out at zero cost.