TypeScript is an increasingly popular way to write modern JavaScript applications. We introduced support for TypeScript in v3.4 of KeyLines, our graph visualization SDK, complete with a full set of definition files.
Whether you’re new to TypeScript or a die-hard convert, this blog post will show you how to use TypeScript with KeyLines to improve your development experience.
What is TypeScript?
TypeScript is a scripting language that compiles into regular JavaScript. Think of it as a way to write cleaner, faster, safer JavaScript code.
JavaScript was designed to be a client-side scripting language for web pages. As JavaScript applications got bigger, it became harder to manage code and scale development. To solve this problem, Microsoft created TypeScript: a strongly typed, open source, superset of JavaScript.
TypeScript works with your existing JavaScript code and runs on any browser, host, or operating system. You can use it with any JavaScript library, and it’s recommended by certain framework vendors, including Angular. It integrates with popular build tools, including Webpack, MSBuild and NuGet, and supports the latest versions of JavaScript (ES6 or ES2015) which use promises instead of callbacks.
Not only does TypeScript plug into your existing JavaScript toolchain, but it also comes with its own powerful tools, including:
- Integration with your IDE’s intelligent code completion and documentation services, to help you read and write code faster and more accurately
- A compiler that transforms TypeScript into standards-compliant JavaScript, including ES6 and ES2015
- Inline error detection at development time and detailed error messages at compile time to help you write better quality code
- Extra language features not in ES6, including enums and the ability to initialize member variables in a constructor
Because it’s open source, developer communities are creating new TypeScript tools all the time. For the latest updates, see What’s New in TypeScript.
How does TypeScript work?
TypeScript is based on a simple, lightweight type system that works with interfaces, classes, arrays and functions. You can use simple annotations to specify data types for variables, parameters and functions, as well as defining interfaces that describe different types of objects.
You can add these type annotations to TypeScript (.ts) files. For example:
// Define a ‘Person’ interface interface Person { firstName: string, lastName: string } // Create an instance of a Person let user : Person = { firstName: “Joe”, lastName: “Bloggs” } // Declare a function which defines a parameter of type Person function sayHello(user: Person) { console.log(“Hello, “ + user.firstName); }
When you run the TypeScript compiler, the output is a JavaScript file.
Type annotations are optional. You don’t have to add them everywhere, so if you’re migrating to TypeScript, you can do so one file at a time without having to do one massive rewrite. You’ll need to save the original .js file as a .ts file so you can add annotations before compiling.
The KeyLines TypeScript definition file
Many popular libraries and frameworks provide definition files that declare their interfaces in TypeScript. These definition files can be used by IDEs and the TypeScript compiler to validate that the libraries are being used correctly. See definitelytyped.org.
We’ve created one for KeyLines that contains the types you need to include in your TypeScript project. Here’s an extract:
interface ChartOptions { /** Controls the appearance of arrows on links. Possible values are 'normal' and 'small'. Default: 'normal'. */ arrows?: "normal" | "small", /** The rgb colour to use for the background of the chart itself. */ backColour?: string,
Notice that the definition file includes API Reference documentation, so you can get point-of-need help as you’re referencing the KeyLines library.
KeyLines type definitions have signatures for the promises version of the KeyLines API. Calls to the callback version still work, but they’re not defined in the types file.
How to use TypeScript with KeyLines
If you don’t have TypeScript already, install it as a Node.js package. At the command line, run:
npm install -g typescript
Alternatively, install Visual Studio Code – a free code editor built using TypeScript.
If you’re using KeyLines 3.4 or later, you’ve already downloaded the KeyLines definition file /ts/keylines.d.ts. Use your IDE to move the .ts file to the folder where your TypeScript project will live.
Now let’s create your first TypeScript file. Make a copy of a JavaScript file in your KeyLines project, save it with a .ts extension.
Update the .ts file with a reference to the KeyLines definition file:
// <reference path=”./keylines.d.ts” />
Use a KeyLines static type to declare a constant:
declare const KeyLines: KeyLines.KeyLines;
Let’s add some simple type annotations to the JavaScript in our new .ts file. Create a function to set the color of an item once it’s selected:
setSelectionColour(colour) { const options = { selectionColour: colour } this.chart.options(options); }
We want the SelectionColour function to be called with a single string parameter and to set chart options:
setSelectionColour(colour: string) { const options: KeyLines.ChartOptions = { selectionColour: colour, }; this.chart.options(options); }
We’ve increased the amount of code, but it’s made the existing calls reliable and error-proof.
Now let’s run the compiler:
tsc.ts
The TypeScript compiler checks that types are being used correctly by cross-referencing against the KeyLines definition file. If the file compiles successfully, the output will be.js file that matches the original JavaScript content.
Now we’ve added a type annotation to our file, we can start taking advantage of TypeScript tooling. Let’s look at a couple of the most popular ones: error reporting, and IDE support for TypeScript code completion.
Reporting errors
We’ll add an error to our setSelectionColour function to see what happens. Let’s change the function parameter type from a string to a number:
setSelectionColour(colour: number) { let options: KeyLines.ChartOptions = { selectionColour: colour, }; this.chart.options(options); }
TypeScript throws an error telling us what’s wrong:
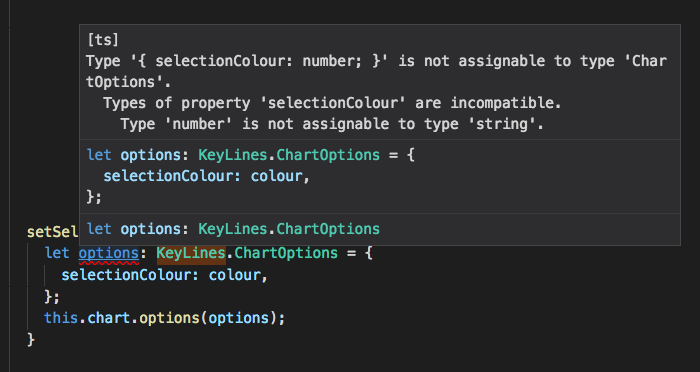
If we compile without fixing it, we’ll get the same error message directly from the TypeScript compiler:
'Type '{ selectionColour: number; }' is not assignable to type 'ChartOptions'. Types of property 'selectionColour' are incompatible. Type 'number' is not assignable to type 'string'.'
Using code completion
Your IDE comes with several features designed to help you write code more quickly and learn more about it. This example shows Microsoft Visual Studio Code’s support, using IntelliSense. It provides context-sensitive information about a function, then prompts you to choose from a list of options:
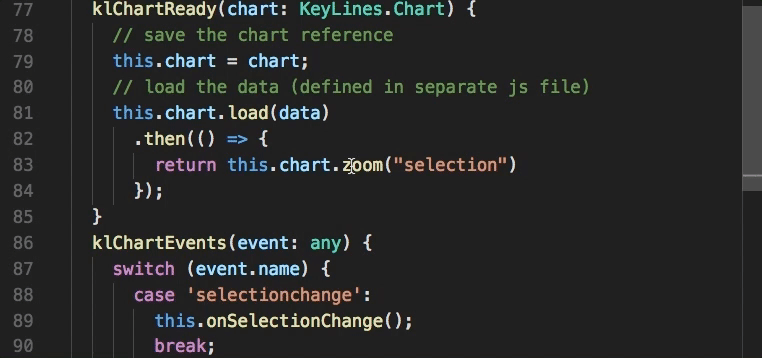
Want to try it for yourself?
This post gives you a taste of the advantages of using TypeScript with KeyLines. Once you start a trial, you’ll be able to access the TypeScript we’ve used as source code for our interactive demos.